Units Of Work

In the realm of software development, particularly within the context of enterprise applications and data-intensive systems, the Unit of Work (UoW) pattern emerges as a pivotal concept for managing database transactions and ensuring data integrity. This pattern provides a way to group one or more operations (often database interactions) into a single transaction, ensuring that all operations either succeed or fail as a single unit. Below, we explore the Unit of Work pattern in depth, examining its purpose, implementation, benefits, and real-world applications.
What is a Unit of Work?
A Unit of Work is a design pattern that maintains a list of objects affected by a business transaction and coordinates the writing out of changes and the resolution of concurrency problems. It acts as a transactional boundary, ensuring that all database operations within its scope are treated as a single, atomic unit. If any operation fails, the entire transaction is rolled back, preserving the consistency of the data.
The Unit of Work pattern is often used in conjunction with the Repository Pattern, where repositories handle CRUD operations, and the Unit of Work manages the transaction scope.
Key Concepts and Components
Transaction Management:
The UoW ensures that all operations within its scope are executed within a single database transaction. This guarantees atomicity—either all operations succeed, or none do.Tracking Changes:
The UoW keeps track of changes (inserts, updates, deletes) made to entities during the transaction. This is crucial for synchronizing in-memory objects with the database.Concurrency Control:
It handles concurrency issues, such as optimistic or pessimistic locking, to prevent data inconsistencies when multiple users modify the same data simultaneously.Commit and Rollback:
The UoW provides methods to commit changes to the database or roll them back in case of failure, ensuring data integrity.
Why Use the Unit of Work Pattern?
- Data Integrity: Ensures atomicity and consistency of database operations.
- Decoupling: Separates transaction management from business logic, improving code modularity.
- Reusability: Provides a standardized way to manage transactions across different parts of an application.
- Concurrency Handling: Simplifies managing concurrent access to data.
- Complexity: Implementing UoW can add complexity, especially in distributed systems.
- Performance Overhead: Tracking changes and managing transactions may introduce performance costs.
Implementation of Unit of Work
Implementing the Unit of Work pattern typically involves the following steps:
- Define the Unit of Work Interface:
Create an interface that defines methods for registering changes, committing, and rolling back transactions.
public interface IUnitOfWork
{
void BeginTransaction();
void Commit();
void Rollback();
IRepository<TEntity> Repository<TEntity>() where TEntity : class;
}
- Implement the Unit of Work Class:
Provide a concrete implementation that manages the transaction scope and coordinates repositories.
public class UnitOfWork : IUnitOfWork
{
private readonly DbContext _context;
private IDbContextTransaction _transaction;
public UnitOfWork(DbContext context)
{
_context = context;
}
public void BeginTransaction()
{
_transaction = _context.Database.BeginTransaction();
}
public void Commit()
{
try
{
_context.SaveChanges();
_transaction?.Commit();
}
catch
{
Rollback();
throw;
}
}
public void Rollback()
{
_transaction?.Rollback();
}
public IRepository<TEntity> Repository<TEntity>() where TEntity : class
{
return new Repository<TEntity>(_context);
}
}
- Integrate with Repositories:
Repositories handle CRUD operations, while the UoW manages the transaction scope.
public class Repository<TEntity> : IRepository<TEntity> where TEntity : class
{
private readonly DbContext _context;
public Repository(DbContext context)
{
_context = context;
}
public void Add(TEntity entity)
{
_context.Set<TEntity>().Add(entity);
}
public void Update(TEntity entity)
{
_context.Set<TEntity>().Update(entity);
}
public void Delete(TEntity entity)
{
_context.Set<TEntity>().Remove(entity);
}
}
Real-World Applications
Case Study: E-Commerce Order Processing
In an e-commerce system, processing an order involves multiple steps: deducting inventory, creating an order record, and updating customer details. Using the Unit of Work pattern ensures that all these operations are treated as a single transaction. If any step fails (e.g., insufficient inventory), the entire transaction is rolled back, preventing inconsistent data.
Unit of Work in Different Technologies
Technology | Implementation Details |
---|---|
.NET (Entity Framework) | Uses DbContext and DbTransaction for transaction management. |
Java (Hibernate) | Leverages Session and Transaction objects for UoW functionality. |
Node.js (Sequelize) | Uses Transaction API to manage database operations as a unit. |
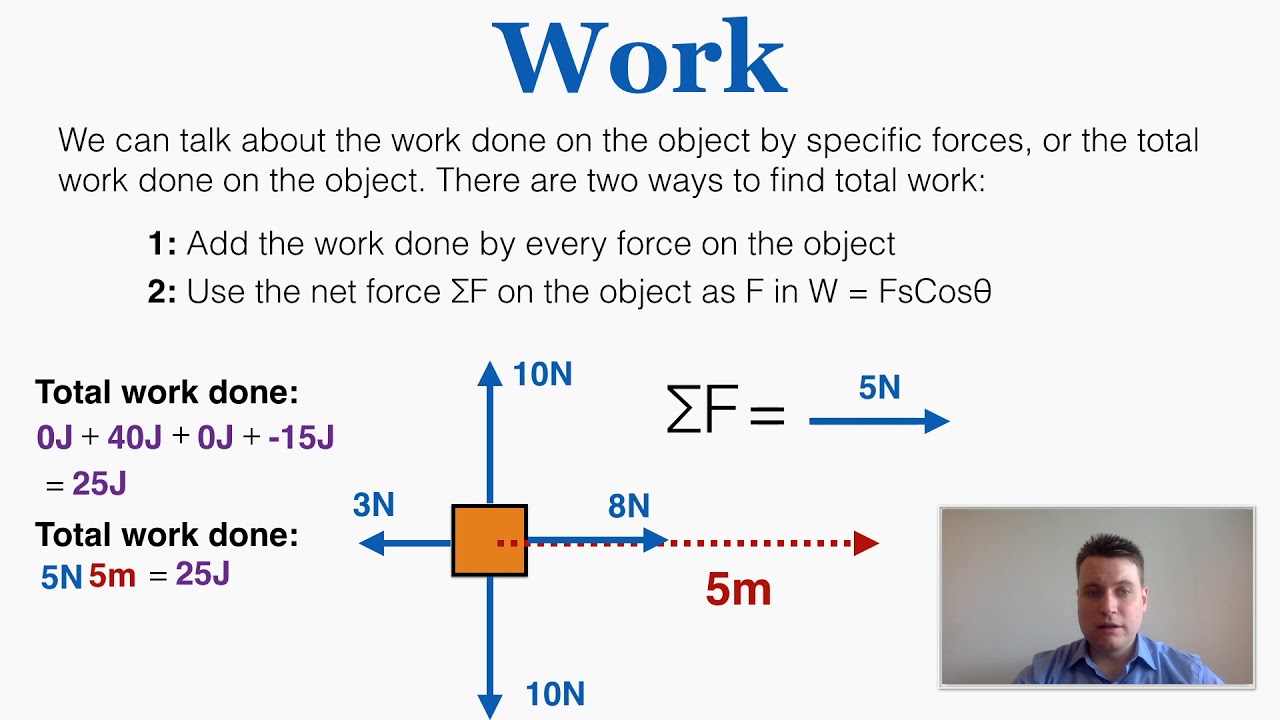
Best Practices
- Keep Transactions Short: Long-running transactions can lead to locks and performance issues.
- Use Isolation Levels Wisely: Choose the appropriate isolation level to balance consistency and performance.
- Handle Exceptions Gracefully: Always roll back transactions when exceptions occur.
- Avoid Chatty Interfaces: Minimize the number of database calls within a transaction.
Future Trends
As distributed systems and microservices architectures become more prevalent, the Unit of Work pattern is evolving to handle distributed transactions. Technologies like SAGA patterns and Two-Phase Commit (2PC) are being integrated with UoW to manage transactions across multiple services.
The rise of event-driven architectures and eventual consistency models may reduce the reliance on traditional UoW patterns, but its core principles remain essential for ensuring data integrity in critical systems.
What is the difference between Unit of Work and Repository Pattern?
+The Repository Pattern is responsible for CRUD operations on a specific entity, while the Unit of Work manages the transaction scope across multiple repositories, ensuring atomicity and consistency.
Can Unit of Work be used in a microservices architecture?
+Yes, but distributed transactions introduce complexity. Patterns like SAGA or event sourcing are often used alongside UoW to manage consistency across services.
How does Unit of Work handle concurrency?
+UoW can implement optimistic or pessimistic locking to handle concurrency. For example, it can check for changes in the database before committing updates.
Is Unit of Work necessary for small applications?
+For small applications with simple data operations, UoW might be overkill. However, it’s beneficial for applications requiring transactional integrity and scalability.
Conclusion
The Unit of Work pattern is a cornerstone of modern software development, providing a robust framework for managing database transactions and ensuring data integrity. By encapsulating transactional logic and coordinating changes, it simplifies complex operations and promotes clean, maintainable code. Whether you’re building a monolithic application or a distributed system, understanding and implementing the Unit of Work pattern can significantly enhance the reliability and scalability of your software.
The Unit of Work pattern is not just about managing transactions—it’s about ensuring that your application’s data remains consistent, even in the face of complexity and failure.